Im schnelllebigen Bereich der künstlichen Intelligenz entwickelt sich die Modellquantisierung zu einer bahnbrechenden Strategie, um große neuronale Netze, insbesondere solche, die große Sprachmodelle (LLMs) antreiben, kontrollierbarer und effizienter zu machen. Bei dieser Methode wird die Genauigkeit der Modelle angepasst, was sich direkt auf die Größe und den Verarbeitungsbedarf des Modells auswirkt. Wir haben aus soliden experimentellen Erkenntnissen gelernt, dass eine hohe Präzision für bestimmte Prozesse in neuronalen Netzen zwar entscheidend ist, andere Prozesse jedoch mit einer geringeren Präzision (z. B. float16) ebenso effizient ablaufen können, was zu einer geringeren Modellgröße führt. Dadurch können diese verkleinerten Modelle auf weniger leistungsfähiger Hardware ausgeführt werden, ohne dass dies zu Lasten der Leistung oder Genauigkeit führt.
In diesem Blog-Beitrag werden wir die Kernelemente der LLM-Quantisierung erörtern.
Was ist Quantisierung?
Im Zusammenhang mit LLMs ist Quantisierung der Prozess zur Umwandlung der kontinuierlichen, unendlichen Werte von Modellgewichten in eine kleinere Menge diskreter, endlicher Werte. Dies wird in der Regel durch die Konvertierung von Gewichten von Datentypen höherer Präzision (wie Float64) in Typen niedrigerer Präzision (wie Float16 oder sogar ganze Zahlen) erreicht. In diesem Zusammenhang bezieht sich der Begriff „Genauigkeit“ darauf, wie viel Speicherplatz in Bits jede Zahl in einem Modell einnimmt. Eine höhere Genauigkeit bedeutet eine höhere Speicherauslastung und im Allgemeinen eine höhere erforderliche Verarbeitungsleistung.
Die Rolle der Genauigkeit in neuronalen Netzen
LLMs sind einfach große neuronale Netze, die Gewichte verwenden, die als Tensoren (mehrdimensionale Arrays aus ganzen Zahlen) im GPU- oder RAM-Speicher gespeichert sind. Die Genauigkeit dieser Faktoren hat einen direkten Einfluss auf den Speicherbedarf und die erforderlichen Verarbeitungsressourcen. Eine höhere Genauigkeit, wie z. B. Float64, verbessert die Trainingsgenauigkeit und Stabilität, erfordert aber mehr Ressourcen. Im Gegensatz dazu kann eine geringere Genauigkeit den Speicherbedarf und den Rechenaufwand verringern und die Operationen effizienter machen.
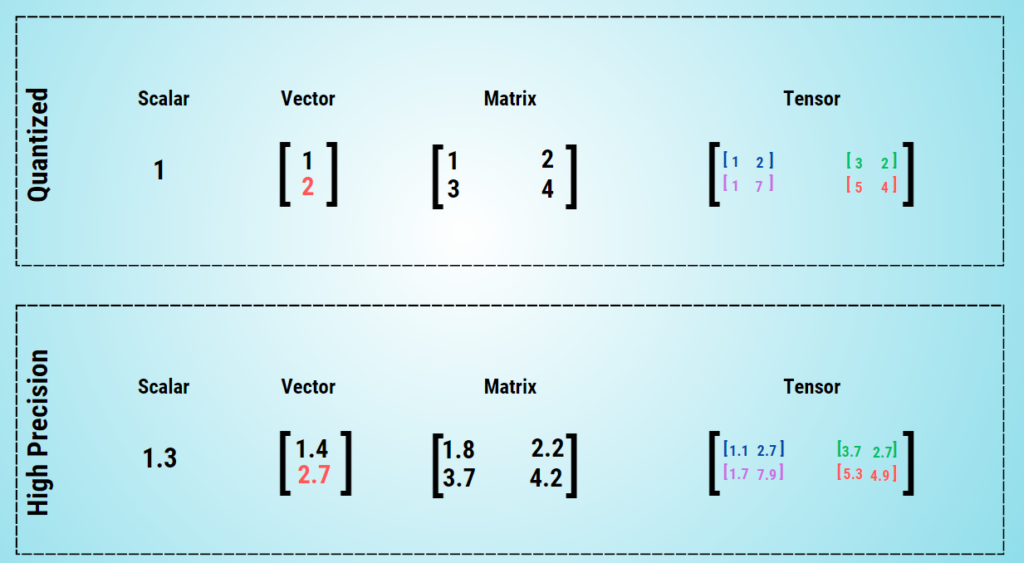
Die Forschung von Computer-Giganten wie Google und Nvidia hat bewiesen, dass eine geringere Genauigkeit für einige Funktionen neuronaler Netze effektiv genutzt werden kann, ohne die Leistung drastisch zu verringern. Die T4-Beschleuniger von Nvidia und die Entwicklung des Datentyps bfloat16 durch Google sind Beispiele für Verbesserungen, die darauf abzielen, Vorgänge mit geringerer Genauigkeit effizienter zu machen. Diese Entwicklungen sind von entscheidender Bedeutung für die kostengünstigere und schnellere Bereitstellung großer Modelle.
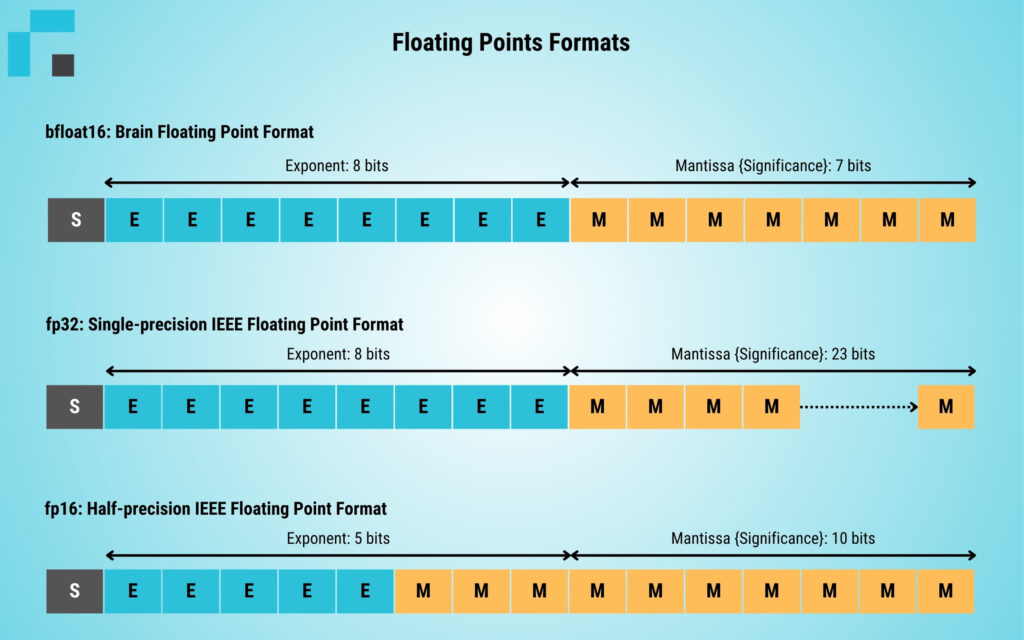
Praktische Implikationen: Eine Fallstudie zur LLM-Quantisierung
Nehmen wir den Grafikprozessor A100 von Nvidia, der als Top-Spezifikation 80 GB RAM bietet. Ein großes Modell wie der Llama2-70B benötigt normalerweise etwa 138 GB RAM. Ohne Quantisierung würde das Hosting dieses Modells zusätzliche A100-GPUs erfordern, was zu höheren Infrastruktur- und Betriebskosten führen würde. Eine quantisierte Version dieses Modells würde dagegen nur etwa 40 GB benötigen, was in eine einzige A100-Einheit passen würde und erhebliche Kosteneinsparungen mit sich brächte. Darüber hinaus würde das quantisierte Modell in dieser Konfiguration Operationen schneller ausführen, was die Leistungseffizienz erhöht.
Model | Originalgröße (FP16) | Quantisierte Größe (INT4) |
Llama2-7B | 13.5 GB | 3.9 GB |
Llama2-13B | 26.1 GB | 7.3 GB |
Llama2-70B | 138 GB | 40.7 GB |
Einfluss der Quantisierung auf die Modellleistung
Durch die Quantisierung wird der Speicherbedarf erheblich reduziert, da weniger Bits für jedes Gewicht benötigt werden, so dass die Modelle auf preiswerterer Hardware und mit höherer Geschwindigkeit ausgeführt werden können. Dies kann sich jedoch auf die Qualität des Modells auswirken. Größere Modelle behalten ihre Effizienz auch bei geringerer Genauigkeit, und einige Untersuchungen deuten darauf hin, dass die 4-Bit-Quantisierung nur einen geringen Einfluss auf die Leistung hat.
Arten von Quantisierungstechniken
Post-Training Quantization (PTQ): Bei dieser Methode wird ein bereits trainiertes Modell in ein Modell mit niedrigerer Genauigkeit umgewandelt. Sie ist einfacher und schneller, kann aber die Leistung leicht beeinträchtigen.
Quantisierungsorientiertes Training (QAT): Bei QAT wird der Quantisierungsprozess in die Trainingsphase des Modells integriert, was häufig zu einer besseren Leistung führt, jedoch höhere Rechenkosten verursacht.
Wahl zwischen einem größeren quantifizierten Modell und einem kleineren nicht quantifizierten Modell
Bei der Optimierung von Leistung und Kosten kann es schwierig sein, zwischen einem kleineren Modell mit voller Genauigkeit und einem größeren quantifizierten Modell zu wählen. Die Ergebnisse der jüngsten Meta-Forschung legen jedoch eine differenzierte Sichtweise nahe. Die Ergebnisse deuten jedoch darauf hin, dass größere quantifizierte Modelle unter ähnlichen Inferenzkostenbedingungen kleinere nicht quantifizierte Modelle tatsächlich übertreffen können.
Dieser Leistungsvorteil ist besonders bei größeren Modellen bemerkenswert, bei denen die Auswirkungen der geringeren Genauigkeit weniger nachteilig sind und eine bessere Leistung, geringere Latenzzeiten und einen höheren Durchsatz ermöglichen. Bei vergleichbaren Kosten kann es daher vorteilhafter sein, ein größeres quantisiertes Modell zu wählen, insbesondere für Anwendungen, die große Rechenressourcen erfordern.
Zugriff auf bereits quantisierte Modelle von Hugging Face Hub
Für diejenigen, die an der Verwendung von quantisierten Modellen interessiert sind, ist der Hugging Face Hub eine Fundgrube. Hier finden Sie verschiedene Modelle, die mit unterschiedlichen Methoden wie GPTQ vorquantisiert wurden und mit Frameworks wie ExLLama oder NF4 kompatibel sind. Ein prominenter Mitwirkender, der auf der Plattform unter dem Namen TheBloke bekannt ist, hat eine Reihe von Modellen mit verschiedenen Quantisierungstechniken veröffentlicht, die es den Nutzern ermöglichen, die beste Lösung für bestimmte Anwendungen auszuwählen.
Einrichtung der Umgebung
Um mit diesen quantisierten Modellen zu experimentieren, können Sie Google Colab verwenden, eine kostenlose GPU-Laufzeitumgebung. Im Folgenden finden Sie die Schritte und den Code, um Ihre Umgebung einzurichten:
1 – Installieren Sie die erforderlichen Bibliotheken: Vergewissern Sie sich zunächst, dass Sie alle erforderlichen Bibliotheken installiert haben. Dazu gehören die Hugging Face Transformator-Bibliothek und zusätzliche Bibliotheken für die spezifische Quantisierungsmethode, die im Modell verwendet wird.
!pip install transformers
!pip install accelerate
# Install libraries for GPTQ quantization method
!pip install optimum
!pip install auto-gptq
2 – Starten Sie die Runtime neu: Nach der Installation kann es notwendig sein, die Runtime in Ihrem Google Colab neu zu starten, um sicherzustellen, dass alle installierten Pakete korrekt geladen werden.
3 – Laden des quantisierten Modells: Sobald die Umgebung eingerichtet ist, kann mit dem Laden des quantisierten Modells fortgefahren werden. Unten sehen Sie ein Beispiel für das Laden eines Llama-2-7B-Chat-Modells, das mit Auto-GPTQ quantisiert wurde:
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
# Define the model ID from Hugging Face Hub
model_id = "TheBloke/Llama-2-7b-Chat-GPTQ"
# Initialize the tokenizer and model with the specified model ID
tokenizer = AutoTokenizer.from_pretrained(model_id, torch_dtype=torch.float16, device_map="auto")
model = AutoModelForCausalLM.from_pretrained(model_id, torch_dtype=torch.float16, device_map="auto")
Dieses Codeschnipsel zeigt Ihnen die Vorbereitung Ihrer Umgebung und das Laden eines vorquantisierten Modells mit Hilfe der speziellen Auto-GPTQ-Methode. Wenn Sie diese Schritte befolgen, können Sie mit quantisierten Modellen experimentieren, um deren Leistung und Anwendbarkeit für verschiedene Aufgaben zu verstehen.
Quantisierung eines beliebigen Modells mit AutoGPTQ und der Transformatorenbibliothek
Obwohl im Hugging Face Hub eine große Auswahl an quantisierten Modellen zur Verfügung steht, gibt es Szenarien, in denen Sie ein Modell selbst quantisieren müssen, entweder weil ein bestimmtes Modell nicht in quantisierter Form verfügbar ist oder weil Sie ein Modell benötigen, das auf eine bestimmte Domäne zugeschnitten ist. Im Folgenden wird beschrieben, wie man ein Modell mit Hilfe der AutoGPTQ-Methode von Optimum – einem Teil von Hugging Face zur Optimierung des Modelltrainings und der Inferenz – manuell quantisieren kann.
Einrichten der Umgebung für die Modellquantisierung
Um mit der Quantisierung zu beginnen, müssen Sie Ihr Modell und die Quantisierungskonfiguration einrichten. In diesem Beispiel verwenden wir ein relativ kleines Modell, um die Konfiguration zu demonstrieren:
from transformers import AutoModelForCausalLM, AutoTokenizer, GPTQConfig
# Specify the model ID for the base model you wish to quantize
model_id = "facebook/opt-125m"
# Initialize the tokenizer for the model
tokenizer = AutoTokenizer.from_pretrained(model_id)
# Define the quantization configuration
quantization_config = GPTQConfig(bits=4, dataset="c4", tokenizer=tokenizer)
# Load the model with the specified quantization configuration
model = AutoModelForCausalLM.from_pretrained(model_id, device_map="auto", quantization_config=quantization_config)
Diese Struktur verwendet eine 4-Bit-Quantisierung, die im Allgemeinen einen guten Kompromiss zwischen Modellgröße und Leistung darstellt. Der Datensatz „c4“ wird hier wegen seiner Vielfalt und Größe verwendet, die für allgemeine Sprachmodelle geeignet sind.
Überlegungen und mögliche Herausforderungen
Die Quantisierung eines Modells, insbesondere eines großen Modells mit 175 B Parametern, kann sehr ressourcenintensiv und zeitaufwendig sein – oft sind mehrere Stunden GPU-Rechenzeit erforderlich. Die Anpassung der Anzahl der Bits oder die Änderung des Datensatzes kann die Leistung des Modells erheblich beeinflussen. Um die Leistung nach der Quantisierung zu maximieren, ist es von Vorteil, einen Datensatz zu wählen, der den Daten, auf die das Modell während der Inferenz trifft, sehr ähnlich ist.
Ihr quantisiertes Modell zu Hugging Face beisteuern
Wenn Sie sich entscheiden, ein Modell zu quantisieren, sollten Sie Ihre Arbeit mit der größeren Gemeinschaft teilen, indem Sie sie im Hugging Face Hub veröffentlichen:
from huggingface_hub import notebook_login, Repository
# Log in to Hugging Face using your account credentials
notebook_login()
# Create a repository for the model and push it
repo = Repository("opt-125m-gptq-4bit", clone_from="your_username/opt-125m-gptq-4bit")
repo.push_to_hub()
Umgang mit Leistungseinbußen
Manchmal kann die Quantisierung eines Modells, insbesondere bei aggressiven Werten oder kleineren Modellen, zu Leistungseinbußen führen. Wenn dies der Fall ist, kann es hilfreich sein, die Eingabeaufforderungen anzupassen, um das Modell effizienter zu steuern, was manchmal dazu beitragen kann, die Genauigkeit und Qualität der Ausgabe aufrechtzuerhalten.
Bemerkenswerte Quantisierungstechniken
Die Modellquantisierung hat sich mit verschiedenen fortgeschrittenen Techniken weiterentwickelt, die die Effizienz und Leistung verbessern. Einige wichtige Methoden sind:
- GPTQ: Diese Technik umfasst Varianten wie AutoGPTQ und GPTQ-for-LLaMa, die sich auf eine effiziente GPU-Ausführung konzentrieren.
- NF4: NF4 wurde unter Verwendung der bitsandbytes-Bibliothek implementiert und in die Hugging-Face-Transformatoren integriert. Es wird häufig in Verbindung mit QLoRA-Methoden verwendet, um Modelle mit einer Genauigkeit von 4 Bit zu verfeinern.
- GGML: GGML ist eine C-Bibliothek, die mit llama.cpp gekoppelt ist und ein einzigartiges Binärformat unterstützt, das das Laden von Modellen beschleunigt und den Zugriff vereinfacht. Die kürzlich erfolgte Umstellung auf das GGUF-Format verbessert auch die Erweiterbarkeit und zukünftige Kompatibilität.
Jede Quantisierungsstrategie, z. B. 4-Bit, 5-Bit und 8-Bit, bietet ein ausgewogenes Verhältnis zwischen Effizienz und Rechenleistung.
Modellquantisierung mit GPTQ
Mit der Entwicklung von GPTQ wurde ein bahnbrechender Ansatz auf dem Gebiet der Modellquantisierung eingeführt, insbesondere für Generative Pre-Trained Transformers. Diese Methode kombiniert auf geschickte Weise die GPT-Modelllinie mit der Post-Training-Quantisierung (PTQ) und passt den Prozess so an, dass große Modelle effizient angepasst werden können. Die Hauptvorteile von GPTQ werden im Folgenden beschrieben:
- Scalability: GPTQ ist in der Lage, riesige Netze, z.B. GPT-Modelle mit bis zu 175 Milliarden Parametern, mit einer Genauigkeit von nur 3 oder 4 Bit pro Gewicht zu komprimieren. Diese Komprimierung wird mit einem minimalen Genauigkeitsverlust erreicht, und das Papier betont, dass der Leistungsunterschied zwischen FP16 und GPTQ umso geringer wird, je größer das Modell ist.
- Performance: Mit GPTQ können Inferenzaufgaben für ein Modell mit 175 Milliarden Parametern auf einem einzigen Grafikprozessor ausgeführt werden, was eine erhebliche Steigerung der Recheneffizienz bedeutet.
- Inference Speed: Auf High-End-Grafikprozessoren wie dem NVIDIA A100 kann GPTQ bis zu 3,25-mal schnellere Inferenzgeschwindigkeiten liefern als FP16-Modelle. Bei preiswerteren Grafikprozessoren wie dem NVIDIA A6000 kann der Geschwindigkeitszuwachs bis zu 4,5 Mal betragen.
GPTQ quantisiert Modelle speziell in INT-basierte Datentypen, meist 4INT. Bei der Integration mit Plattformen wie ExLLama können mit 4-Bit-GPTQ quantisierte Modelle noch höhere GPU-Beschleunigungen erreichen. Die Integration von GPTQ mit AutoGTPQ von Hugging Face verwendet standardmäßig ExLLama, um die Beschleunigung zu verbessern.
ExLLama: Optimiert für moderne GPUs
ExLLama ist eine spezielle Anpassung der Llama-Bibliothek für die Verwendung mit 4-Bit-GPTQ-Gewichten. Sie wurde für hohe Leistung und geringen Speicherbedarf auf modernen GPUs entwickelt und ist daher ideal für neuere Hardware. Die Einführung von ExLlamaV2 verspricht weitere Verbesserungen, obwohl es sich noch in einem frühen Entwicklungsstadium befindet.
Praktische Anwendung der GPTQ
Die GPTQ-Quantisierung kann auf eine Vielzahl von Modellen angewendet werden und ermöglicht die Umwandlung in 3-, 4- oder 8-Bit-Darstellungen in einfachen Schritten. AutoGPTQ, das sich durch seine nahtlose Integration in die Transformator-Bibliothek auszeichnet, ist nach wie vor das beliebteste Werkzeug für die Implementierung der GPTQ-bezogenen Quantisierung. In den vorangegangenen Abschnitten wurde ein detailliertes Beispiel für die Verwendung von AutoGPTQ für die Quantisierung und die effektive Verwendung von quantisierten Modellen gegeben.
NF4 und doppelte Quantisierung mit Bitsandbytes zur Modellkompression
Der Datentyp NormalFloat (NF), insbesondere NF4 (4-Bit NormalFloat), stellt eine Weiterentwicklung der Quantilsquantisierungstechnik dar. Er liefert bessere Ergebnisse als herkömmliche 4-Bit Ganzzahlen und Fließkommazahlen. In Verbindung mit der Doppelquantisierung (DQ) erreicht NF4 höhere Kompressionsraten ohne Leistungseinbußen. Der DQ-Ansatz besteht aus zwei Phasen: zunächst die Verarbeitung der Quantisierungskonstanten und dann die Verwendung dieser Konstanten für die weitere Quantisierung zur Erzeugung von FP32- und FP8-Werten. Diese Methode spart effektiv Speicherplatz – etwa 0,37 Bit pro Parameter, was bei großen Modellen wie einem 65B-Parametermodell von Bedeutung ist.
Die kürzlich erfolgte Aufnahme dieser Techniken in die bitsandbytes-Bibliothek, wie im QLoRA-Papier erwähnt, hat gezeigt, dass die 4-Bit-Quantisierung sowohl in der Inferenz- als auch in der Trainingsphase für große Sprachmodelle (LLMs) effektiv und ohne Leistungseinbußen eingesetzt werden kann.
Hier erfahren Sie, wie Sie die bitsandbytes-Bibliothek für NF4 und Doppelquantisierung verwenden können, die nahtlos in die Hugging-Face-Transformator-Bibliothek integriert ist:
# Install the bitsandbytes library
!pip install bitsandbytes
from transformers import AutoModelForCausalLM, AutoTokenizer, BitsAndBytesConfig
import torch
# Configure the NF4 settings with Double Quantization
nf4_config = BitsAndBytesConfig(
load_in_4bit=True,
bnb_4bit_quant_type="nf4",
bnb_4bit_use_double_quant=True,
bnb_4bit_compute_dtype=torch.bfloat16
)
# Specify the model name
model_name = "PY007/TinyLlama-1.1B-step-50K-105b"
# Initialize the tokenizer with the NF4 configuration
tokenizer_nf4 = AutoTokenizer.from_pretrained(model_name, quantization_config=nf4_config)
# Load the model with the NF4 configuration
model_nf4 = AutoModelForCausalLM.from_pretrained(model_name, quantization_config=nf4_config)
Bitte beachten Sie, dass das Quantisieren und Laden von großen Modellen sehr ressourcenintensiv sein kann und viel RAM benötigt. Das Beispiel ist jedoch so konzipiert, dass es auf einem kostenlosen Google Colab GPU läuft.
Darüber hinaus unterstützt die bitsandbytes-Bibliothek eine einzigartige Funktion, mit der Modellgewichte zwischen CPU und GPU aufgeteilt werden können. Die Gewichte auf der CPU bleiben in float32 und werden nicht in 8-Bit konvertiert, was bei der Handhabung großer Modelle von Vorteil sein kann, da die Last zwischen GPU- und CPU-Ressourcen ausgeglichen wird. Diese Funktion hilft bei der effizienten Verwaltung großer Modelle, ohne den GPU-Speicher zu überlasten, und ermöglicht die Ausführung großer Modelle auf leichter zugänglichen Hardwarekonfigurationen.
GGML und llama.cpp
Im dynamischen Bereich des maschinellen Lernens nimmt GGML eine einzigartige Stellung ein. GGML, benannt nach den Initialen seines Schöpfers Georgi Gerganov, ist eine C-Bibliothek, die sich auf die effiziente Verarbeitung von Aufgaben des maschinellen Lernens konzentriert. Ihre Einzigartigkeit liegt in ihrem proprietären Binärformat, das ursprünglich entwickelt wurde, um LLMs auf eine Weise zu verteilen, die sich von herkömmlichen Formaten unterscheidet.
Übergang zum GGUF-Format
Eine wichtige Entwicklung in der GGML-Entwicklung ist der Übergang zum GGUF-Format, das eine breitere Palette von Modellen unterstützen soll als das ursprüngliche Lama-zentrierte Format. GGUF wurde entwickelt, um erweiterbar und zukunftssicher zu sein und um den RAM-Bedarf während der Quantisierung erheblich zu reduzieren, wodurch es ideal für eine Vielzahl von Computerumgebungen ist.
Integration mit der llama.cpp Bibliothek
Die Bibliothek llama.cpp ergänzt GGML, indem sie die Verwendung von LLaMA-Modellen erleichtert, wobei der Schwerpunkt auf der 4-Bit-Integer-Quantisierung liegt. Ihr Hauptziel ist es, die Verwendung dieser Modelle auf alltäglichen Geräten wie MacBooks zu ermöglichen, indem sie für einen geringen Ressourcenverbrauch bei gleichbleibender Leistung optimiert wird.
Verbesserte Funktionalität für verschiedene Hardware
Ursprünglich konnten mit GGML Modelle direkt auf CPUs geladen und ausgeführt werden. Neuere Erweiterungen erlauben es jedoch, bestimmte Rechenschritte auf GPUs auszulagern. Dies beschleunigt nicht nur die Inferenz, sondern ermöglicht auch die Ausführung großer LLMs, die normalerweise die durchschnittliche VRAM-Kapazität übersteigen würden.
Consumer-Hardware mit beeindruckender Inferenzleistung
Jüngste Entwicklungen haben gezeigt, dass ein 180B Falcon-Modell unter Verwendung von GGML und llama.cpp Inferenzaufgaben auf einem Mac M2 Ultra ausführen kann. Dies ist ein bemerkenswerter Beweis dafür, wie die Quantisierung selbst die größten Modelle auf verbraucherfreundlicher Hardware zugänglich machen kann.
Quantisierung einer LLM für CPU-Inferenz
Die Quantisierung eines großen Sprachmodells (LLM) für eine effiziente CPU-Inferenz umfasst mehrere detaillierte Schritte. Für diejenigen, die an der Anwendung dieser Techniken interessiert sind, wird hier eine Vorgehensweise vorgeschlagen:
- 1 – Lesen Sie eine ausführliche Anleitung, die Sie Schritt für Schritt durch den GGML- und llama.cpp-Quantisierungsprozess führt.
- 2 – Alternativ kann ein Skript aus dem Repository llama.cpp verwendet werden, um ein beliebiges Hugging Face-Modell einfach zu quantisieren.
Abschließende Überlegungen zur Quantisierung
Fortschritte in der Quantisierung haben die Nutzung großer Sprachmodelle verändert und leistungsstarke KI-Funktionen ohne Leistungseinbußen auf Alltagsgeräten verfügbar gemacht. Diese Demokratisierung der KI-Technologie ermöglicht einem größeren Nutzerkreis den Zugang zu fortschrittlichen Sprachverarbeitungswerkzeugen und fördert so eine breitere Akzeptanz und Innovation.
Die laufenden Entwicklungen in GGML und llama.cpp sind ein Beispiel für das Engagement der KI-Gemeinschaft, Spitzentechnologie für ein breites Spektrum von Anwendern zugänglich und nutzbar zu machen. Da sich dieser Bereich weiterentwickelt, sind weitere Innovationen zu erwarten, die die Lücke zwischen der Spitzenforschung im Bereich der KI und der Technologie für die breite Öffentlichkeit weiter schließen werden.
Lesen Sie außerdem:
https://www.skillbyte.de/ki-in-der-logistik-revolutionierung-der-branche/
https://www.skillbyte.de/advanced-ai-applications-for-the-insurance-domain/